블로그 이사했습니다!
👇 블로그 이전 공지 👇
👇 새 블로그에서 글 보기 👇
[Android] 이미지 받아 다른 액티비티에 전달하기 — Win Record (tistory.com)
[Android] 이미지 받아 다른 액티비티에 전달하기
⚠️ 2021.01.14에 작성된 글입니다 ⚠️ 코드는 [Android] 이미지 가져오기 - 카메라, 갤러리 이용 에서 이어집니다. 화면 - activity_get_image.xml 이미지를 카메라 또는 갤러리에서 받아오는 화면 <?xml ver
win-record.tistory.com
코드는 이미지 가져오기 관련 첫 글에서 이어집니다.
화면
- activity_get_image.xml
이미지를 카메라 또는 갤러리에서 받아오는 화면
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".GetImageActivity"> <ImageView android:id="@+id/iv_main" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/btn_camera" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="5dp" android:text="@string/camera" /> <Button android:id="@+id/btn_gallery" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="5dp" android:text="@string/gallery" /> <Button android:id="@+id/btn_move" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="5dp" android:text="@string/move" /> </LinearLayout> </LinearLayout>
- activity_set_image.xml
받아온 이미지 세팅하는 화면
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".SetImageActivity"> <TextView android:id="@+id/tv_image_path" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:padding="10dp" android:textColor="@color/black" android:textSize="20sp" /> <ImageView android:id="@+id/iv_image" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1" /> </LinearLayout>
이미지 받아오는 액티비티
- GetImageActivity.java
public class GetImageActivity extends AppCompatActivity implements View.OnClickListener { final int CAMERA = 100; // 카메라 선택시 인텐트로 보내는 값 final int GALLERY = 101; // 갤러리 선택 시 인텐트로 보내는 값 String imagePath = ""; @SuppressLint("SimpleDateFormat") SimpleDateFormat imageDate = new SimpleDateFormat("yyyyMMdd_HHmmss"); Intent intent; ImageView imageView; Button btnCamera, btnGallery, btnMove; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_get_image); imageView = findViewById(R.id.iv_main); btnCamera = findViewById(R.id.btn_camera); btnGallery = findViewById(R.id.btn_gallery); btnMove = findViewById(R.id.btn_move); btnCamera.setOnClickListener(this); btnGallery.setOnClickListener(this); btnMove.setOnClickListener(this); // 권한 체크 boolean hasCamPerm = checkSelfPermission(Manifest.permission.CAMERA) == PackageManager.PERMISSION_GRANTED; boolean hasWritePerm = checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE) == PackageManager.PERMISSION_GRANTED; if (!hasCamPerm || !hasWritePerm) // 권한 없을 시 권한설정 요청 ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.CAMERA, Manifest.permission.WRITE_EXTERNAL_STORAGE}, 1); } @SuppressLint({"NonConstantResourceId", "QueryPermissionsNeeded"}) @Override public void onClick(View view) { switch (view.getId()) { case R.id.btn_camera: // 카메라 선택 시 intent = new Intent(android.provider.MediaStore.ACTION_IMAGE_CAPTURE); if (intent.resolveActivity(getPackageManager()) != null) { File imageFile = null; try { imageFile = createImageFile(); } catch (IOException e) { e.printStackTrace(); } if (imageFile != null) { Uri imageUri = FileProvider.getUriForFile(getApplicationContext(), "com.example.sendimage.fileprovider", imageFile); intent.putExtra(MediaStore.EXTRA_OUTPUT, imageUri); startActivityForResult(intent, CAMERA); } } break; case R.id.btn_gallery: // 갤러리 선택 시 intent = new Intent(Intent.ACTION_PICK); intent.setType(MediaStore.Images.Media.CONTENT_TYPE); intent.setType("image/*"); startActivityForResult(intent, GALLERY); break; case R.id.btn_move: // 이동 선택 시 if (imagePath.length() > 0) { // 이미지 경로가 있을 경우 intent = new Intent(getApplicationContext(), SetImageActivity.class); intent.putExtra("path", imagePath); startActivity(intent); } break; } } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (resultCode == Activity.RESULT_OK) { // 결과가 있을 경우 if (requestCode == GALLERY) { // 갤러리 선택한 경우 // 1) data의 주소 사용하는 방법 imagePath = data.getDataString(); // "content://media/external/images/media/7215" // 2) 절대경로 사용하는 방법 Cursor cursor = getContentResolver().query(data.getData(), null, null, null, null); if (cursor != null) { cursor.moveToFirst(); int index = cursor.getColumnIndex(MediaStore.Images.ImageColumns.DATA); imagePath = cursor.getString(index); // "/media/external/images/media/7215" cursor.close(); } } if (imagePath.length() > 0) { Glide.with(this) .load(imagePath) .into(imageView); } } } @SuppressLint("SimpleDateFormat") File createImageFile() throws IOException { // 이미지 파일 생성 String timeStamp = imageDate.format(new Date()); // 파일명 중복을 피하기 위한 "yyyyMMdd_HHmmss"꼴의 timeStamp String fileName = "IMAGE_" + timeStamp; // 이미지 파일 명 File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES); File file = File.createTempFile(fileName, ".jpg", storageDir); // 이미지 파일 생성 imagePath = file.getAbsolutePath(); // 파일 절대경로 저장하기 return file; } }
갤러리에서 이미지를 받아올 때 1)intent의 정보를 사용하는 것과 2)절대경로 사용하는 방법 모두 다른 액티비티로 이미지 경로를 전달할 때 작동한다.
이미지 전달받는 액티비티비
- SetImageActivity.java
public class SetImageActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_set_image); TextView textView = findViewById(R.id.tv_image_path); ImageView imageView = findViewById(R.id.iv_image); String imagePath = getIntent().getStringExtra("path"); textView.setText(imagePath); Glide.with(this).load(imagePath).into(imageView); } }
결과화면


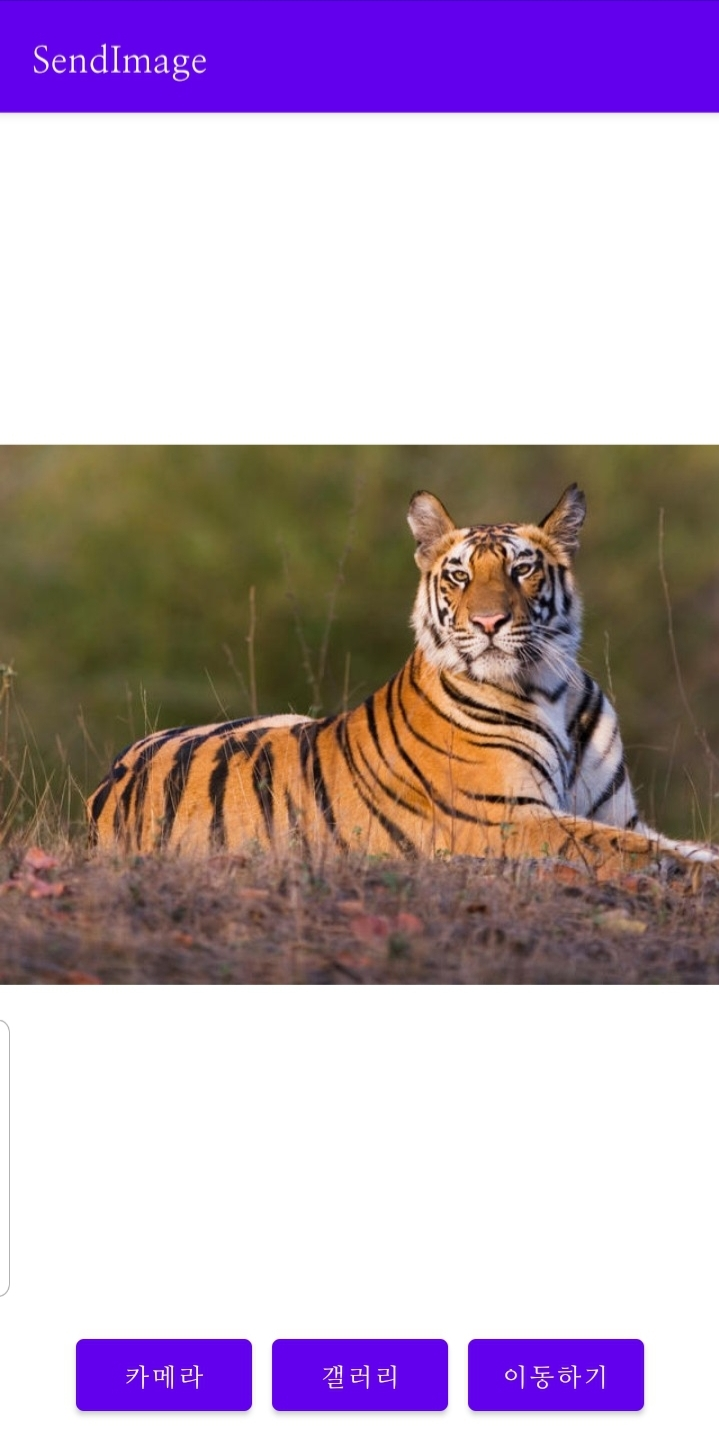

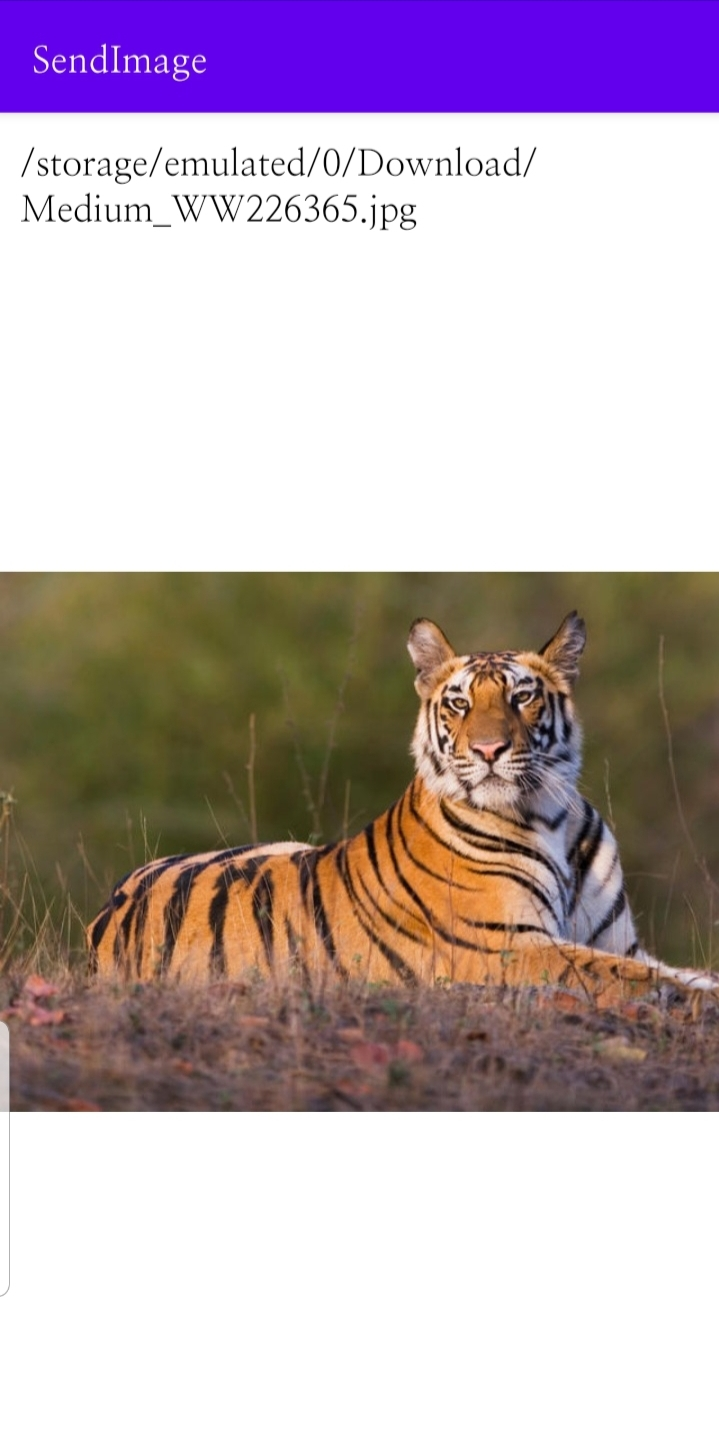
가운데 사진은 1)의 방법으로, 오른쪽 사진은 2)의 방법으로 이미지 경로값을 가져온 것이다.
1) 방법인 data.getDataString()으로는 모든 경로의 파일이 불러와지지만
2) 방법인 Cursor객체를 사용해 절대경로를 가져오는 방법은 특정 폴더에서는 아래 line4의 index에 null이 들어온다. 따라서 현재로서는 1)의 방법을 사용하는게 낫다.
Cursor cursor = getContentResolver().query(data.getData(), null, null, null, null); if (cursor != null) { cursor.moveToFirst(); int index = cursor.getColumnIndex(MediaStore.Images.ImageColumns.DATA); imagePath = cursor.getString(index); // "/media/external/images/media/7215" cursor.close(); }
절대경로를 가져오는 방법에 대해선 다시 더 공부해야 할 필요가 있다.
전체 코드
이전 글
다음 글
Firbase 사진 업로드하기 (tistory.com)
공부하며 정리한 글입니다. 내용에 대한 피드백은 언제나 환영입니다.
'android' 카테고리의 다른 글
SharedPreferences 사용해 데이터 저장하기 (0) | 2021.01.15 |
---|---|
Firbase 사진 업로드하기 (0) | 2021.01.14 |
가져온 이미지 회전 막기 (0) | 2021.01.14 |
Glide 사용하기 (0) | 2021.01.14 |
이미지 가져오기 - 카메라, 갤러리 이용 (1) | 2021.01.14 |
댓글